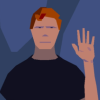
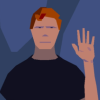
Performance, compilers, hardware, mathematics, computer science.
I've worked in or adjacent to the video game industry for most of my career.
This profile is from a federated server and may be incomplete. Browse more on the original instance.
Performance, compilers, hardware, mathematics, computer science.
I've worked in or adjacent to the video game industry for most of my career.
This profile is from a federated server and may be incomplete. Browse more on the original instance.